
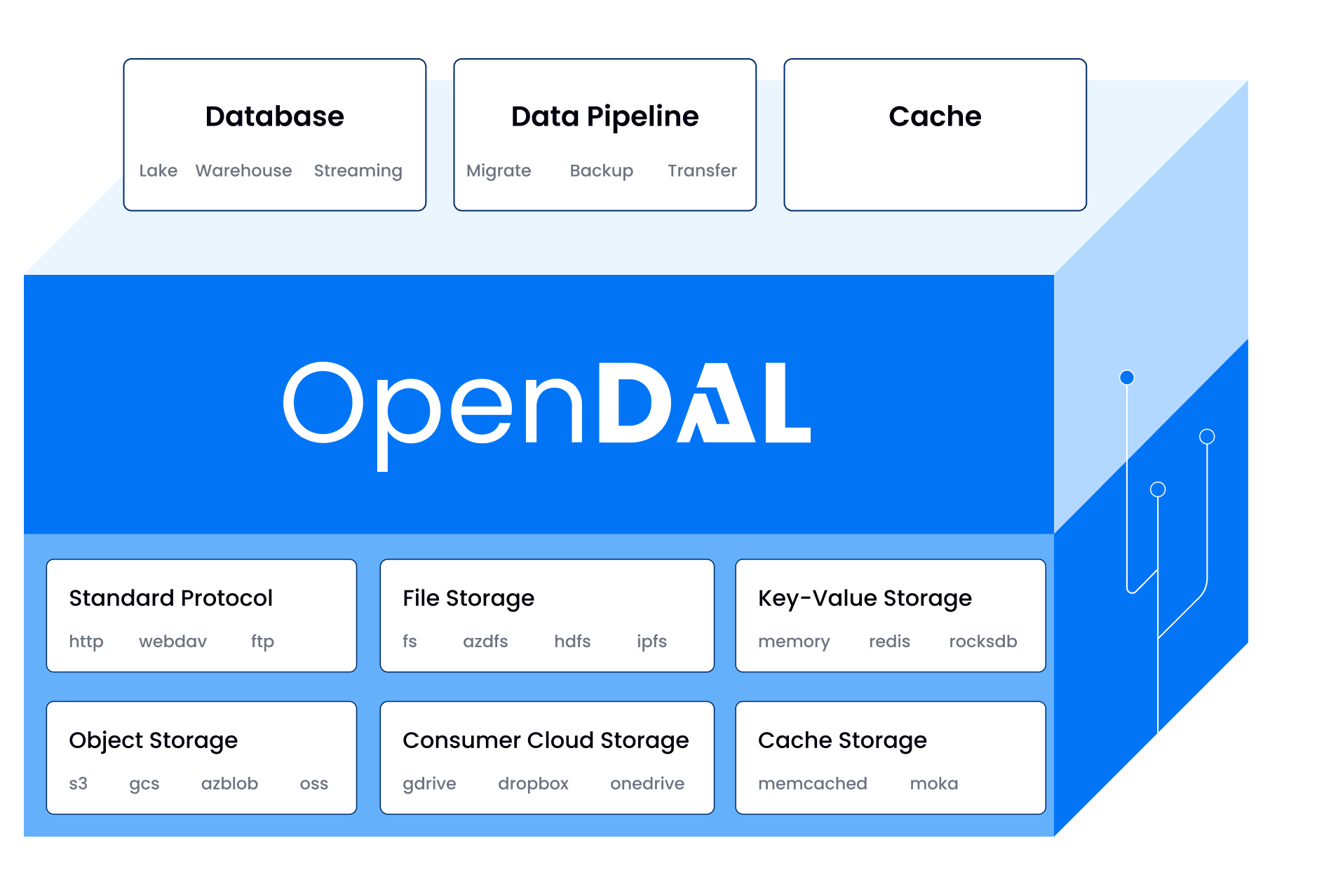
Example
A simple read and write example
#include "assert.h"
#include "stdio.h"
int main()
{
assert(result.operator_ptr != NULL);
assert(result.
error == NULL);
.
data = (uint8_t*)
"this_string_length_is_24",
.len = 24,
};
assert(error == NULL);
assert(read_bytes.
len == 24);
for (int i = 0; i < 24; ++i) {
printf(
"%c", read_bytes.
data[i]);
}
printf("\n");
}
struct opendal_error * opendal_operator_write(const struct opendal_operator *op, const char *path, const struct opendal_bytes *bytes)
Blocking write raw bytes to path.
void opendal_operator_free(const struct opendal_operator *ptr)
Free the heap-allocated operator pointed by opendal_operator.
struct opendal_result_operator_new opendal_operator_new(const char *scheme, const struct opendal_operator_options *options)
Construct an operator based on scheme and options
struct opendal_result_read opendal_operator_read(const struct opendal_operator *op, const char *path)
Blocking read the data from path.
void opendal_bytes_free(struct opendal_bytes *ptr)
Frees the heap memory used by the opendal_bytes.
opendal_bytes carries raw-bytes with its length
Definition opendal.h:99
uintptr_t len
Definition opendal.h:107
uint8_t * data
Definition opendal.h:103
The opendal error type for C binding, containing an error code and corresponding error message.
Definition opendal.h:133
The result type returned by opendal_operator_new() operation.
Definition opendal.h:241
struct opendal_error * error
Definition opendal.h:249
The result type returned by opendal's read operation.
Definition opendal.h:278
struct opendal_bytes data
Definition opendal.h:282
struct opendal_error * error
Definition opendal.h:286
For more examples, please refer to ./examples
Prerequisites
To build OpenDAL C binding, the following is all you need:
- A compiler that supports C11 and C++14, e.g. clang and gcc
- To format the code, you need to install clang-format
- The
opendal.h
is not formatted by hands when you contribute, please do not format the file. Use make format
only.
- If your contribution is related to the files under
./tests
, you may format it before submitting your pull request. But notice that different versions of clang-format
may format the files differently.
- (optional) Doxygen need to be installed to generate documentations.
For Ubuntu and Debian:
# install C/C++ toolchain
sudo apt install -y build-essential
# install clang-format
sudo apt install clang-format
# install and build GTest library under /usr/lib and softlink to /usr/local/lib
sudo apt-get install libgtest-dev
# install CMake
sudo apt-get install cmake
# install Rust
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Makefile
To build the library and header file.
mkdir -p build && cd build
cmake ..
make
- The header file
opendal.h
is under ./include
- The library is under
../../target/debug
after building.
- use
FEATURES
to enable services, like cmake .. -DFEATURES="opendal/services-memory,opendal/services-fs"
To clean the build results.
cargo clean
cd build && make clean
To build and run the tests. (Note that you need to install Valgrind and GTest)
cd build
make tests && ./tests
To build the examples
cd build
make basic error_handle
Documentation
The documentation index page source is under ./docs/doxygen/html/index.html
. If you want to build the documentations yourself, you could use
# this requires you to install doxygen
make doc
Used by
Check out the users list for more details on who is using OpenDAL.
License and Trademarks
Licensed under the Apache License, Version 2.0: http://www.apache.org/licenses/LICENSE-2.0
Apache OpenDAL, OpenDAL, and Apache are either registered trademarks or trademarks of the Apache Software Foundation.